2009
May
26
Cookie
Tags:
What are cookies?
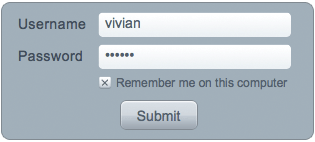
Cookies are small amounts of data stored by the web browser. They allow you to store particular information about a user and retrieve it every time they visit your pages. Each user has their own unique set of cookies.
Cookies are typically used by web servers to perform functions such as tracking your visits to websites, enabling you to log in to sites, and storing your shopping cart.
document.cookie
Cookie
- document.cookie = "name=value; expires=date; path=path; domain=domain; secure";
Property | Description |
---|---|
name=value | This sets both the cookie's name and its value. |
expires=date | This optional values sets the date that the cookie will expire on. The date should be in the format returned by the toGMTString() method of the Date object. If the expires value is not given, the cookie will be destroyed the moment the browser is closed. |
path=path | This optional value specifies a path within the site to which the cookie applies. Only documents in this path will be able to retrieve the cookie. Usually this is left blank, meaning that only the path that set the cookie can retrieve it. |
domain=domain | This optional value specifies a domain within which the cookie applies. Only websites in this domain will be able to retrieve the cookie. Usually this is left blank, meaning that only the domain that set the cookie can retrieve it. |
secure | This optional flag indicates that the browser should use SSL when sending the cookie to the server. This flag is really used. |
Set a Cookie
Cookie
- var COOKIE = {
- set: function(name, value, options) {
- if (!name) return;
- options = options || {};
- if (!options.expires) options.expires = 1;
- var date = new Date();
- date.setTime(date.getTime() + options.expires*24*60*60*1000);
- var expires = "; expires=" + date.toGMTString();
- var path = options.path ? '; path=' + (options.path) : '';
- var domain = options.domain ? '; domain=' + (options.domain) : '';
- var secure = options.secure ? '; secure' : '';
- document.cookie = [name, "=", encodeURIComponent(value), expires, path, domain, secure].join("");
- }
- };
Retrive a Cookie
Example
- var COOKIE = {
- get: function(name) {
- if (!name) return;
- var value = "";
- if (document.cookie && document.cookie != "") {
- var cookies = document.cookie.split(";");
- for (var i=0; i<cookies.length; i++) {
- var cookie = cookies[i];
- // Trim the space.
- while(cookie.charAt(0) == " ") cookie = cookie.substring(1, cookie.length);
- // Tell if this cookie string begins with the name we want.
- if (cookie.substring(0, name.length + 1) == (name + "=")) {
- value = decodeURIComponent(cookie.substring(name.length + 1));
- break;
- }
- }
- }
- return value;
- }
- };
Delete a Cookie
Example
- <code>
- var COOKIE = {
- del: function(name) {
- if (!name) return;
- this.set(name, "", -1);
- }
- };
- </code>