2014
Mar
14
Overview
Based on the library bootstrap model and jstree, I built the library Treeview to select a file from the file cabinet and do copy or move.
Getting Started
Step 1: Include CSS and JavaScript of Libraries
Example
- <link rel="stylesheet" href="../libraries/bootstrap-3.1.1/dist/css/bootstrap.css">
- <link rel="stylesheet" href="/static/css/common/miii-modals.css">
- <script src="../libraries/jquery/jquery-1.10.1.js"></script>
- <script src="../libraries/bootstrap-3.1.1/dist/js/bootstrap.min.js"></script>
- <script src="../libraries/jstree-v.pre1.0/jquery.jstree.js"></script>
- <!-- jquery.nicescroll.js is optional. It's included for customzied scroll bar. -->
- <script src="../libraries/jquery.nicescroll.340/jquery.nicescroll.js"></script>
Step 2: Include CSS and JavaScript of Treeview
Example
- <link rel="stylesheet" href="css/treeview.css">
- <script src="js/treeview_lang.js"></script>
- <script src="js/treeview.js"></script>
Samples
Select Single Directory
Example
- var treeview = new Treeview({
- isDirOnly : true,
- isCreateDir : true,
- isShow : true,
- title : "從檔案櫃選擇",
- subtitle : "所有檔案櫃",
- desc : "把資料搬移到:",
- buttonLabel : "搬移",
- langs : MIIICLOUD.lang,
- callback : function (result) {
- if (result.action === "ok") {
- console.log(result.selectedData);
- console.log(result.createdData);
- }
- }
- });
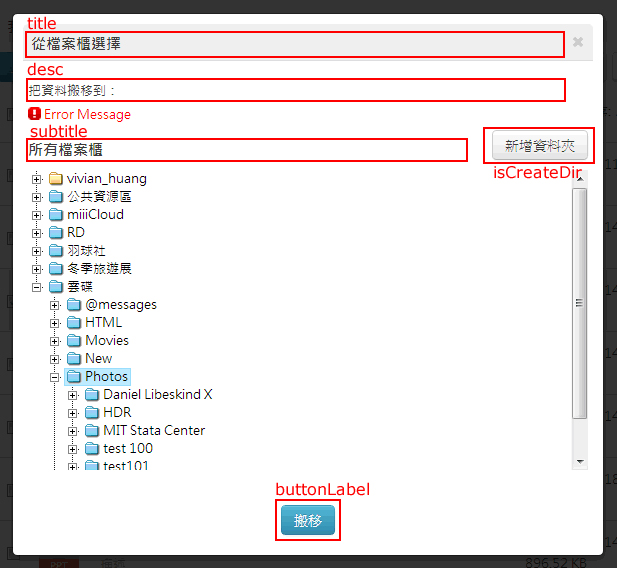
Select Multiple Files
Example
- var treeview = new Treeview({
- gid : 14,
- isFileMulti : true,
- title : "從檔案櫃選擇",
- desc : "可勾選單一或多個檔案,然後再發佈於訊息牆中",
- buttonLabel : "確定",
- isShow : true,
- langs : MIIICLOUD.lang,
- callback : function (result) {
- if (result.action === "ok") {
- console.log(result.selectedData);
- console.log(result.createdData);
- }
- }
- });
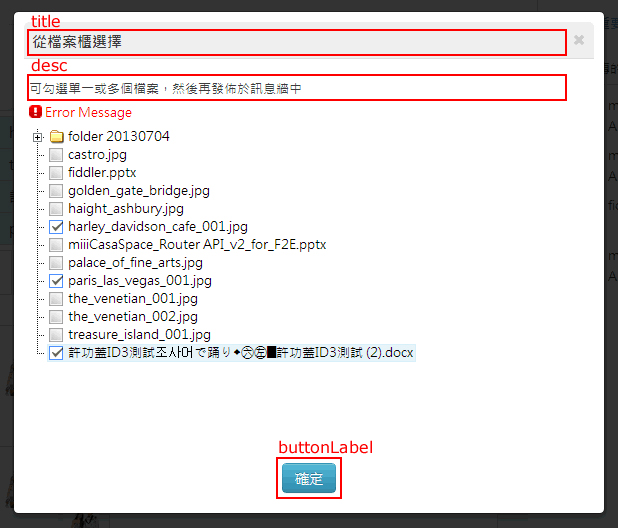
Select Single File
Example
- var treeview = new Treeview({
- isShow : true,
- title : "從檔案櫃選擇",
- desc : "勾選一個檔案,然後開始編輯",
- buttonLabel : "確定",
- langs : MIIICLOUD.lang,
- callback : function (result) {
- console.log(result.selectedData);
- console.log(result.createdData);
- }
- });
Libraries
Bootstrap Modal HTML Markup
Example
- <div id="treeview-modal-1374552592089" class="modal fade">
- <div class="modal-dialog">
- <div class="modal-content">
- <div class="modal-header">
- <a href="#" class="close" data-dismiss="modal"><i class="icon-remove"></i></a>
- <h3>Treeview</h3>
- </div>
- <div class="modal-body">
- <div class="miii-treeview">
- <div class="empty hide">
- <p class="main"></p>
- <p></p>
- </div>
- <div class="outer-content hide">
- <div>
- <p class="desc"></p>
- <p class="msg hide"></p>
- <h4 class="pull-left"></h4>
- <button class="btn pull-right hide">Create Folder</button>
- </div>
- <div class="inner-content">
- <div id="treeview-1374552592089"></div>
- </div>
- </div>
- </div>
- </div>
- <div class="modal-footer">
- <button class="btn btn-primary">Ok</button>
- </div>
- </div>
- </div>
- </div>
jstree Config
Example
- $('#treeview-1374552592089').jstree({
- 'plugins': [
- 'themes', 'json_data', 'ui', 'types', 'crrm'
- ],
- 'themes': {
- 'theme' : 'miii',
- 'dots' : true,
- 'icons' : true
- },
- 'ui': {
- 'selected_parent_close': 'select_parent'
- },
- // This will prevent moving or creating any other type as a root node
- 'valid_children': ['group'],
- 'types' : {
- // can have files and other folders inside of it
- 'valid_children': ['group'],
- 'types': {
- 'folder': {
- 'icon': {
- 'image': '/static/lib/jstree-v.pre1.0/themes/miii/ico_folder.png'
- }
- },
- 'user-folder': {
- 'icon': {
- 'image': '/static/lib/jstree-v.pre1.0/themes/miii/ico_folder_user.png'
- }
- }
- }
- },
- 'json_data': {
- 'ajax': {
- 'url': '/api/files/getFileList',
- 'correct_state': true,
- // the `data` function is executed in the instance's scope
- // the parameter is the node being loaded
- // (may be -1, 0, or undefined when loading the root nodes)
- 'data': function (n) {
- if (n === -1) {
- return {
- 'gid' : 14,
- 'dironly' : 0,
- 'permission' : 1, // permission 1: viewable, permission 2: uploadable.
- 'format' : 'jstree',
- };
- } else {
- return {
- 'gid' : n.data('gid'),
- 'path' : n.data('path'),
- 'dironly' : 0,
- 'permission' : 1,
- 'format' : 'jstree'
- };
- }
- }
- }
- }
- });
jstree Render HTML Markup
Example
- <div id="treeview-1374552592089" class="jstree jstree-0 jstree-focused jstree-miii">
- <ul>
- <li data-name="@km" data-gid="14" data-path="/@km" data-size="4096" data-crumb="805802324b3a95c4e810450c586cdcac" class="folder jstree-closed jstree-unchecked" rel="user-folder">
- <ins class="jstree-icon"> </ins>
- <a href="#">
- <ins class="jstree-checkbox"> </ins>
- <ins class="jstree-icon"> </ins>知識庫的檔案
- </a>
- </li>
- <li data-name="castro.jpg" data-gid="14" data-path="/USA Trip" data-size="130452" data-crumb="" class="file jstree-leaf jstree-unchecked" rel="user-folder">
- <ins class="jstree-icon"> </ins>
- <a href="#" class="">
- <ins class="jstree-checkbox"> </ins>
- <ins class="jstree-icon"> </ins>castro.jpg
- </a>
- </li>
- </ul>
- </div>
API
Treeview Config
Parameter | Required | Type | Default | Description |
---|---|---|---|---|
buttonLabel | No | String | Ok | Treeview button value. |
callback | No | Function | function () {} | Function will be triggered when clicking on the ok button or hiding the treeview model. |
desc | No | String | "" | Treeview descriptions. |
gid | No | Number | 0 | What directory to start getting data from? |
isCreateDir | No | Boolean | false | To provide the feature to create a new directory. |
isDirMulti | No | Boolean | false | To provide the feature to allow multi-selection of directories. |
isDirOnly | No | Boolean | false | To get data of directories only. |
isFileMulti | No | Boolean | false | To provide the feature to allow mulit-selection of files. |
isShow | No | Boolean | false | Show treeview in the beginning. |
langs | No | Object | {} | Treeview international lang kyes. |
subtitle | No | String | "" | Treeview subtitle. |
title | No | String | Treeview | Treeview model title. |
Example
- var treeview = new Treeview({
- gid : 0, // List tree from this group id. if 0, list from root node.
- isDirMulti : false, // Allow directories to be multiple selected.
- isFileMulti : true, // Allow files to be multiple selected.
- isDirOnly : false, // List directories only, no files.
- isCreateDir : false, // Show the button of create folder.
- isShow : false, // Show the modal on the initial.
- title : '從檔案櫃選擇', // The title of treeview.
- subtitle : '', // The subtitle of treeview.
- desc : '', // The description of treeview.
- buttonLabel : '確定', // The button label of treeview.
- langs : {}, // The lang object of treeview.
- callback : function (result) { // The callback function is triggered when closing the treeview modal.
- console.log(result.action); // action === 'ok' or action === 'close'
- console.log(result.selectedData); // The selected items.
- console.log(result.createdData); // The directories created.
- }
- });
Callback
result.action
Click on the ok button or hide the treeview model.
Example
- if (result.action === "ok") {
- // after clicking on the ok button
- // continue your code here
- } else if (result.action === "close") {
- // click on the modal close button
- }
result.selectedData
The selected directories or files.
Example
- result.selectedData = [
- {
- "filename" : "新增資料夾",
- "filesize" : 0,
- "gid" : 14,
- "path" : "/新增資料夾"
- }
- ];
result.createdData
The directories users created.
Example
- result.createdData = [
- {
- "filename" : "新增資料夾",
- "filesize" : 0,
- "gid" : 14,
- "path" : ""
- },
- {
- "filename" : "新增資料夾 (2)",
- "filesize" : 0,
- "gid" : 14,
- "path" : ""
- }
- ];
Treeview Methods
reset()
Uncheck all directories and files, and close all directories.
Example
- treeview.reset();
show()
Show the treeview model, and uncheck all directories or files and close all directories.
Example
- treeview.show();
hide()
Hide treeview model.
Example
- treeview.hide();
showErrors()
Show error messages
Example
- treeview.showErrors('Error Message', function () {
- // after showing the error message
- // continue your code here
- });
Mockup Data
JSON Data
Example
- [
- {
- "data": {
- "title": "知識庫的檔案", // 顯示在 Treeview 上的目錄或檔案名稱
- "attr": {
- "href": "#"
- }
- },
- "attr": {
- "data-name": "@km", // 實際目錄或檔案名稱
- "data-gid": "14",
- "data-path": "\/@km",
- "data-size": 4096,
- "data-crumb": "f519fc5b76163d4c05bc1d1bc48f2c16",
- "class": "folder", // "folder" or "file" 用來區別 item 為目錄或檔案。
- "rel": "user-folder" // "user-folder" 自己工作區的資料夾 icon,"folder" 其他群組的資料夾 icon。
- },
- "state": "closed"
- },
- {
- "data": {
- "title": "USA Trip",
- "attr": {
- "href": "#"
- }
- },
- "attr": {
- "data-name": "USA Trip",
- "data-gid": "14",
- "data-path": "\/USA Trip",
- "data-size": 4096,
- "data-crumb": "f519fc5b76163d4c05bc1d1bc48f2c16",
- "class": "folder",
- "rel": "user-folder"
- },
- "state": "closed"
- },
- {
- "data": {
- "title": "miiiCloud",
- "attr": {
- "href": "#"
- }
- },
- "attr": {
- "data-name": "miiiCloud",
- "data-gid": 2,
- "data-path": "",
- "data-size": 0,
- "data-crumb": "d3678e423493725a80167833290eea40",
- "class": "folder",
- "rel": "folder"
- },
- "state": "closed"
- },
- {
- "data": {
- "title": "Dinner_At_Grand_Hotel_Palazzo_della_Fonte_In_Fiuggi_004.JPG",
- "attr": {
- "href": "#"
- }
- },
- "attr": {
- "data-name": "Dinner_At_Grand_Hotel_Palazzo_della_Fonte_In_Fiuggi_004.JPG",
- "data-gid": "14",
- "data-path": "",
- "data-size": 498513,
- "data-crumb": "",
- "class": "file",
- "rel": "user-folder"
- },
- "state": ""
- }
- ]
回應 (Leave a comment)